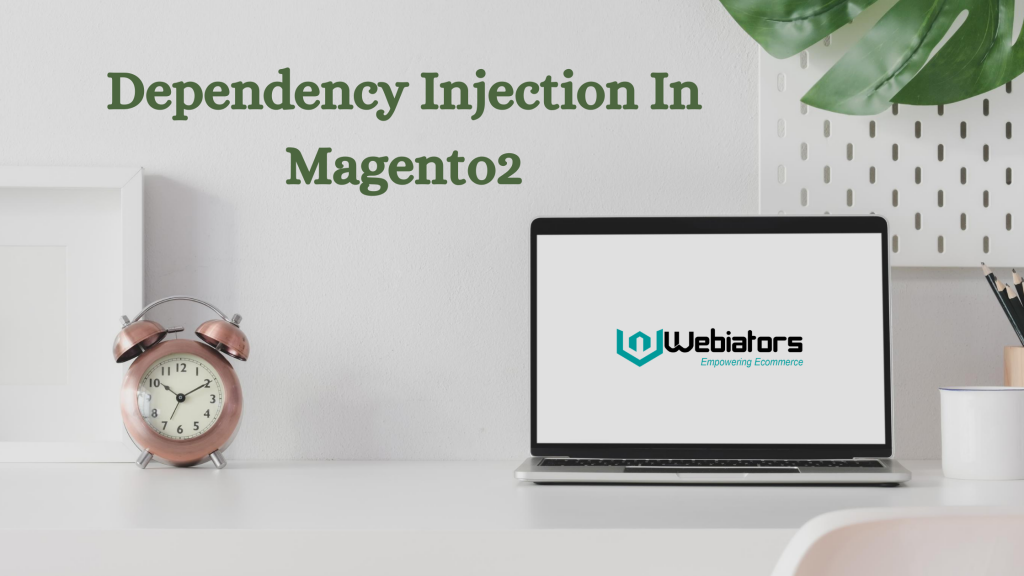
In this blog post, we will try to understand Dependency Injection in Magento 2 as simply as possible.
Lets understand each of them separately:
‘Dependency‘ just means that something is dependent on something like you are dependent on your parents. and ‘Injection’ simply means to provide something or to inject something by a third person.
In programming languages, a dependency is known as the object which is required by a class to execute some functions. The injection is the passing of that particular dependency to a dependent object/class. The original meaning of Dependency Injection is to inject the dependency into the class from another source.
For example –
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 | class Student { private $firstName; private $lastName; public function __construct($firstName, $lastName) $this->lastName = $lastName; { $this->firstName = $firstName; } public function getFirstName() { return $this->firstName; } public function getLastName() { return $this->lastName; } } class StudentInfo { private $student; public function __construct($studentFirstName, $studentLastName) { $this->student = new Student($studentFirstName, $studentLastName); } public function getStudent() { return $this->student; } } |
There is nothing wrong with the above code. It will return the first & last name without any issues. But the Student class is tightly joined with the StudentInfo class. If you add a new parameter to the constructor of the Student class, then you have to change every class where you had created a Student object with the new operator, which subjects it to a slow and lengthy process, especially when it comes to the large applications.
Dependency Injection solves these issues by injecting the dependencies through the dependent class’ constructor. The result is a very maintainable code that can be used in long-term projects. It might look like this:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 | class Student { private $firstName; private $lastName; public function __student($firstName, $lastName) { $this->firstName = $firstName; $this->lastName = $lastName; } public function getFirstName() { return $this->firstName; } public function getLastName() { return $this->lastName; } } class StudentInfo { private $student; public function __construct(Student $student) { $this->student = $student; } public function getStudent() { return $this->student; } } |
In the above code, instead of instantiating dependency with the new operator, we defined it in a __construct parameter. It is known as Automatic Dependency Injection.
That’s it!
If you have any doubts about this topic, please mention them in the comment section below.
Thank you.